Entity
An Entity is an object or a concept for which data is stored, and operations are performed. For example, Vehicle, Box, User, etc.
Entity Category: The type of object or concept about which data is stored and manipulated. For example, four-wheeler or two-wheeler is a category of entity vehicle.
Entity Sub-category: The type of Entity Category for which data is stored and manipulated. For example, a truck or a car is an entity sub-category for the entity category four-wheeler whose entity is vehicle.
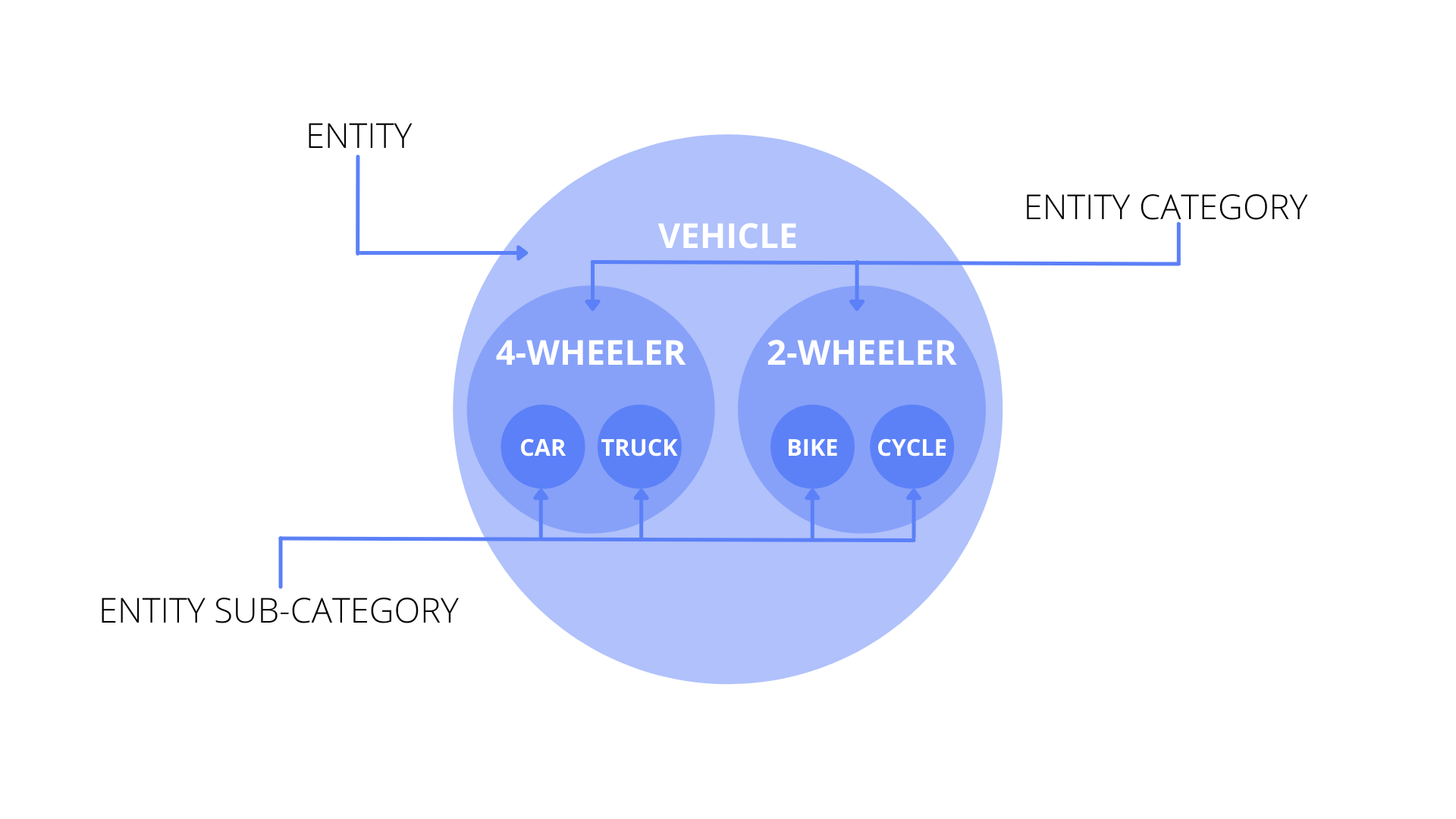
An Entity Template is created based on an entity category-sub-category pair. The attributes created in the entity template override the attributes created for the entity.
An instance of an entity is created either by instantiating an entity or by instantiating an entity template.
🚧 NOTE
Lifecycle rules governing the state of an entity are not in the scope of this document and are covered separately in the State Machine Documentation.
The Entity service exposes API endpoints that allow you to store the data and perform operations on the data for an Entity. It is responsible for managing the availability, latency, consistency, and replication of the data stored.
Using the Entity Service you can:
- Create multiple entities per App. For example, for a ticketing system (App), entities could be users, tickets, vendors, etc.
- Define entities by creating Attributes.
- Expose CRUD APIs.
- Search or filter the stored data.
- Search or filter the changes in attributes and data.
- The batch creates or updates the data.
- Perform templatization of entities and creation of templates based on entity category and sub-category.
- Configure data warehouse capability.
📘 Entity Service new attribute addition
Updates to existing Attributes and the addition of new Attributes take effect after the creation of a new Entity instance and are not backward compatible.
You can create templates that you can use to simplify the creation of Entity instances.
The process to create a template:
- The Entity Instance is created using the Entity Template. The Entity instance has the superset of all the attributes defined in the Entity template and Entity. If attributes with the same name exist in the Entity template and Entity definition, then the Attributes of the Entity template will override the attributes of the Entity.
- If the attributes of the Entity or the Attributes of the Entity template are updated, Entity instances created after the update is complete will have the updated values. Previously created Entity instances are not updated with new Attributes or Attribute updates.
Let's understand how you can use Templatization in a real-world example:
- Create an Entity: We created an entity named vehicle with base and custom attributes. Suppose, vehicle has two custom attributes mileage and manufacturer with default values 15 and Toyota, respectively.
- Create Entity-category and Entity-subcategory: We defined Entity-category for the entity vehicle as four-wheeler and Entity-subcategory is categorized as car.
- Create a template based on the Entity category and the Entity subcategory: We create a template called vehicleTemplate1 for Entity-category four-wheeler and entity-subcategory car.
- Defining Attributes for the Entity Template vehicleTemplate1: We defined two custom Attributes mileage and manufacturer with default values 50 and Suzuki, respectively.
- Now, we have 3 cases for creating Entity instances:
Case 1: When the Entity instance is created using Entity. For example, car123 is created using the entity vehicle. The values of mileage and manufacturer are provided during instance creation hence mileage: 35 and manufacturer: Volkswagen.
Case 2: When the Entity instance is created using Entity. For example, car123 is created using the Entity vehicle. The values for custom Attributes mileage and manufacturer are not provided. In that case, the default values of 15 and Toyota are picked up while creating the instance.
Case 3: When the Entity instance is created using the Entity template vehicleTemplate1. If the values for custom Attributes, mileage, and manufacturer, are provided during instance creation, those values are associated with the instance. However, if the values of mileage and manufacturer are not provided, the default values of vehicleTemplate1 Attributes are picked up overriding the entity values of mileage and manufacturer. In this case, the instance has amileage: 50 and manufacturer: Suzuki stored across the instance.
To create an Entity Template, call the Create Template endpoint. To update an Entity Template, call the Update Template endpoint.
Request bodies are specified in JSON format. The following examples show a request body for creating an Entity Template and a request body for updating an Entity Template:
Member | Description | Validation | Data type |
---|---|---|---|
name | Template name. | pattern: ^[a-z-A-Z0-9][a-zA-Z0-9 ]{0,63}$ | string |
subCategory | Template sub-category. | pattern: ^[a-zA-z][a-zA-z0-9,_\-]{0,15}$ minLength: 1 maxLength: 16 | string |
attributes>name | Attribute name. | minLength: 1 maxLength: 32 pattern: ^[a-zA-Z]{1,32}$ | string |
attributes>description | Attribute description. | minLength: 0 maxLength: 256 | string |
attributes>dataType | Data type. | Valid values: string, number, boolean, object, array, address, phone, money | string |
attributes>indexed | Defines whether to make this attribute indexed or not so that filtering can be provided based on this attribute. | default: false | boolean |
attributes>defaultValue | Provide the default value of the attribute. The data type of the value should match the data type of the attribute defined. | | number |
attributes>validation | Validation rules for a string or number value. | | string |
attributes>required | Specifies whether the value is required or optional. | default: false | boolean |
callback | URL to notify the outcome of the request. Returns event data for success and failure events. | pattern: https?:\/\/(www\.)?[-a-zA-Z0-9@:%.\+~#=]{2,256}\.[a-z]{2,4}\b([-a-zA-Z0-9@:%\+.~#?&//=]*) | string |
To create an Entity Instance, call the Create Entity endpoint. To update an Entity Instance, call the Update Entity endpoint.
Request bodies are specified in JSON format. The following examples show a request body for creating an Entity Instance and a request body for updating an Entity Instance:
Member | Description | Validation | Data type |
---|---|---|---|
uniqueCode | Unicode code for the entity instance. | minLength: 1 maxLength: 128 pattern: ^[a-zA-Z0-9][a-zA-Z0-9_:-]{0,127} | string |
name | Template name. | pattern: ^[a-z-A-Z0-9][a-zA-Z0-9 ]{0,63}$ | string |
subCategory | Template sub-category. | pattern: ^[a-zA-z][a-zA-z0-9,_\-]{0,15}$ minLength: 1 maxLength: 16 | string |
attributes>name | Attribute name. | minLength: 1 maxLength: 32 pattern: ^[a-zA-Z]{1,32}$ | string |
attributes>description | Attribute description. | minLength: 0 maxLength: 256 | string |
attributes>dataType | Data type. | Valid values: string, number, boolean, object, array, address, phone, money | string |
attributes>indexed | Defines whether to make this attribute indexed or not so that filtering can be provided based on this attribute. | default: false | boolean |
attributes>defaultValue | Provide the default value of the attribute. The data type of the value should match the data type of the attribute defined. | | number |
attributes>validation | Validation rules for a string or number value. | | string |
attributes>required | Specifies whether the value is required or optional. | default: false | boolean |
callback | URL to notify the outcome of the request. | pattern: https?:\/\/(www\.)?[-a-zA-Z0-9@:%.\+~#=]{2,256}\.[a-z]{2,4}\b([-a-zA-Z0-9@:%\+.~#?&//=]*) | string |
The create, read, and update endpoints are used to define the Entities per app. Note that, the app name is mandatory when defining an entity.
Entities are identified by their pluralName which must be unique per tenant and app. The Entity pluralName is created as a separate resource so that you can create separate Entity instances. Allowed owners should be an enum that contains the owning entity pluralNames (it is validated during entity creation). If empty, then no validation is performed.
A Tenant can define Custom Attributes for an Entity. The service allows you to:
- Define the name and data type of the attribute.
- Provide optional default value of the attribute.
- Define validations on the attribute. The following validations are allowed:
- Range- Defining min-max.
- ValueOneOf- Homogenous enum (values of the enum should be of the same data type).
- Required- Whether the attribute is required or not.
An Entity is defined by Base and Custom Attributes:
Base Attributes: These are the attributes pre-defined by the platform for the Entity. Some of these attributes are obligatory. Custom Attributes: These are the attributes that the Tenant defines for the Entities, to enhance their usability.
Attribute Type | Attribute Name | Description |
---|---|---|
Base | Tenant Id***** | The ID of the tenant to whose realm the entity belongs. |
Base | Entity Id***** | This is a system-generated ID, expected to be unique within a tenant. |
Base | Category | Category of an entity. |
Base | Sub-category | The sub-category of an entity. |
Base | Template | Template based on which entity instance is created. |
Custom | Attributes 1 to n (Indexed) | Custom attributes for an entity with indexing. |
Custom | Attributes 1 to n (Non-indexed) | Custom attributes for an entity without indexing. |
*isActive, isDeleted, createdAt, createdBy, updatedAt, updatedBy, or any such database columns should be automatically created by the developers.
To create an Entity Type that has Custom Attributes, call the Create Entity Type endpoint. To update an Entity Type, call the Update Entity Type endpoint.
Request bodies are specified in JSON format. The following examples show a request body for creating an Entity Type and a request body for updating an Entity Type:
Member | Description | Validation | Data type |
---|---|---|---|
name>plural | Plural name of the entity type. | pattern: ^[a-zA-Z0-9]{1,16}$ minLength: 1 maxLength: 16 example: vehicles | string |
name>singular | Singular name of entity-type | pattern: ^[a-zA-Z0-9]{1,16}$ minLength: 1 maxLength: 16 example: vehicle | string |
category>name | Category name | example: vehicle pattern: ^[a-zA-z][a-zA-z0-9,_\-]{0,15}$ minLength: 1 maxLength: 16 | string |
category>description | Category description. | | string |
category>subCategory | Sub-category | pattern: ^[a-zA-z][a-zA-z0-9,_\-]{0,15}$ minLength: 1 maxLength: 16 | string |
attribute>name | Attribute name. | minLength: 1 maxLength: 32 pattern: ^[a-zA-Z]{1,32}$ | string |
attribute>description | Attribute description. | minLength: 0 maxLength: 256 | string |
attribute>dataType | The data type of the attribute. | Valid values: string, number, boolean, object, array, address, phone, money, geo-point | string |
attribute>indexed | Defines whether to make this attribute indexed or not so that filtering can be provided based on this attribute. | default: false | boolean |
isStateMachineEnabled | | default: false | boolean |
events | Events. | minItems: 1 maxItems: 999 | string |
entityCode | Entity code. | | string |
The entity_type data type allows you to reference other entities as properties within an entity schema. When creating or updating an entity type, you can include entity_type as a custom attribute:
Filter and search GET endpoints are provided for retrieving entity instances based on various filters like match, multi-match, term, range, regex, exists, prefix, wildcard, etc. The results of the call are paginated. To configure the result set, use the following options: limit, offset, from(time), and to(time).
Batch endpoints are provided to create and update Entities in batches. The Batch endpoints support two methods to supply the Entity data:
- JSON
- CSV file upload
To register a Batch Entity request, call the Create Batch Request endpoint. To update a Batch Entity request, call the Update Batch Request endpoint.
Member | Description | Validation | Data type |
---|---|---|---|
payload | List of valid objects to perform create data in Entities. | maxItems: 1024 minItems: 1 | |
uniqueCode | Unique code. | minLength: 1 maxLength: 128 | string |
name | Name of the Entity. | minLength: 1 maxLength: 64 | string |
owner | The owner of the Entity should be a valid Entity ID. | minLength: 1 maxLength: 64 | string |
category | Valid entity category. | example: vehicle pattern: ^[a-zA-z][a-zA-z0-9,_\-]{0,15}$ minLength: 1 maxLength: 16 | string |
subCategory | Valid entity sub-category. | example: car pattern: ^[a-zA-z][a-zA-z0-9,_\-]{0,15}$ minLength: 1 maxLength: 16 | string |
properties | Key-Value representation of all core attributes of the Entity. | | |
callback | URL to notify the outcome of the request. | pattern: https?:\/\/(www\.)?[-a-zA-Z0-9@:%.\+~#=]{2,256}\.[a-z]{2,4}\b([-a-zA-Z0-9@:%\+.~#?&//=]*) | string |
Request bodies are specified in JSON format. The following examples show a request body for creating a Batch Entity request and a request body for updating a Batch Entity request:
👍 Entity Batch Processing
Container can be created and updated in batch using Batch Processing API.
Here are the sample CSV files for Batch Creation & Updation of Containers.
The lifecycle for the Entities defined in the Entity service is managed separately using the State Machine Service. To use/not use the state machine service for lifecycle management is dependent on the Tenant’s business use case.
Storing the data in the data warehouse is Tenant configurable. By default, the data is only stored in the transactional database. When the data warehouse feature is enabled, the data becomes available in the data warehouse becomes available immediately. It is advisable to build reporting dashboards in the data warehouse.
The Tenant can configure a terminal TTL that governs the availability of data in the transactional database. If the value is not provided, the data is deleted from the transactional database after 30 days. If the data warehouse feature is enabled, then the data is available to query from the DWH after the deletion from the transactional database. If it is not enabled the data is permanently deleted.